// // //----------------------------------------------------------------------------------- // DESCRIPTION : BIN to seven segments converter // segment encoding // a // +---+ // f | | b // +---+ <- g // e | | c // +---+ // d // Enable (EN) active : high // Outputs (data_out) active : low // Download from : http://www.pld.com.cn //----------------------------------------------------------------------------------- module bin27seg (data_in ,EN ,data_out ); input [3:0] data_in ; input EN ; output [6:0] data_out ; reg [6:0] data_out ; always @(data_in or EN ) begin data_out = 7'b1111111; if (EN == 1) case (data_in ) 4'b0000: data_out = 7'b1000000; // 0 4'b0001: data_out = 7'b1111001; // 1 4'b0010: data_out = 7'b0100100; // 2 4'b0011: data_out = 7'b0110000; // 3 4'b0100: data_out = 7'b0011001; // 4 4'b0101: data_out = 7'b0010010; // 5 4'b0110: data_out = 7'b0000011; // 6 4'b0111: data_out = 7'b1111000; // 7 4'b1000: data_out = 7'b0000000; // 8 4'b1001: data_out = 7'b0011000; // 9 4'b1010: data_out = 7'b0001000; // A 4'b1011: data_out = 7'b0000011; // b 4'b1100: data_out = 7'b0100111; // c 4'b1101: data_out = 7'b0100001; // d 4'b1110: data_out = 7'b0000110; // E 4'b1111: data_out = 7'b0001110; // F default: data_out = 7'b1111111; endcase end endmodule 二进制到格雷码转换
// //----------------------------------------------------------------------------------- // DESCRIPTION : Bin to gray converter // Input (DATA_IN) width : 4 // Enable (EN) active : high // // Download from : http://www.pld.com.cn //----------------------------------------------------------------------------------- module BIN2GARY (EN ,DATA_IN ,DATA_OUT ); input EN ; input [3:0] DATA_IN ; output [3:0] DATA_OUT ; assign DATA_OUT [0] = (DATA_IN [0] ^ DATA_IN [1] ) && EN ; assign DATA_OUT [1] = (DATA_IN [1] ^ DATA_IN [2] ) && EN ; assign DATA_OUT [2] = (DATA_IN [2] ^ DATA_IN [3] ) && EN ; assign DATA_OUT [3] = DATA_IN [3] && EN ; endmodule 二进制到BCD码转换// // //----------------------------------------------------------------------------------- // DESCRIPTION : Bin to Bcd converter // Input (data_in) width : 4 // Output (data_out) width : 8 // Enable (EN) active : high // // Download from : http://www.pld.com.cn //----------------------------------------------------------------------------------- module bin2bcd (data_in ,EN ,data_out ); input [3:0] data_in ; input EN ; output [7:0] data_out ; reg [7:0] data_out ; always @(data_in or EN ) begin data_out = {8{1'b0}}; if (EN == 1) begin case (data_in [3:1]) 3'b000 : data_out [7:1] = 7'b0000000; 3'b001 : data_out [7:1] = 7'b0000001; 3'b010 : data_out [7:1] = 7'b0000010; 3'b011 : data_out [7:1] = 7'b0000011; 3'b100 : data_out [7:1] = 7'b0000100; 3'b101 : data_out [7:1] = 7'b0001000; 3'b110 : data_out [7:1] = 7'b0001001; 3'b111 : data_out [7:1] = 7'b0001010; default : data_out [7:1] = {7{1'b0}}; endcase data_out [0] = data_in [0]; end end endmodule 多路选择器(MUX)// // //----------------------------------------------------------------------------------- // DESCRIPTION : Multiplexer // Code style: used case statement // Width of output terminal: 8 // Number of terminals: 4 // Output enable active: HIGH // Output value of all bits when enable not active: 0 // Download from : http://www.pld.com.cn //----------------------------------------------------------------------------------- module mux(EN ,IN0 ,IN1 ,IN2 ,IN3 ,SEL ,OUT ); input EN ; input [7:0] IN0 ,IN1 ,IN2 ,IN3 ; input [1:0] SEL ; output [7:0] OUT ; reg [7:0] OUT ; always @(SEL or EN or IN0 or IN1 or IN2 or IN3 ) begin if (EN == 0) OUT = {8{1'b0}}; else case (SEL ) 0 : OUT = IN0 ; 1 : OUT = IN1 ; 2 : OUT = IN2 ; 3 : OUT = IN3 ; default : OUT = {8{1'b0}}; endcase end endmodule 双向管脚(clocked bidirectional pin)Verilog HDL: Bidirectional Pin This example implements a clocked bidirectional pin in Verilog HDL. The value of OE determines whether bidir is an input, feeding in inp, or a tri-state, driving out the value b. download from: http://www.fpga.com.cn bidir.v module bidirec (oe, clk, inp, outp, bidir); // Port Declaration input oe; input clk; input [7:0] inp; output [7:0] outp; inout [7:0] bidir; reg [7:0] a; reg [7:0] b; assign bidir = oe ? a : 8'bZ ; assign outp = b; // Always Construct always @ (posedge clk) begin b <= bidir; a <= inp; end endmodule
共2条
1/1 1 跳转至页
Verilog HDL 程序举例--基本组合逻辑功能:
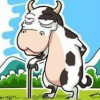
关键词: Verilog 程序 举例 基本 组合 逻辑 功能
共2条
1/1 1 跳转至页
回复
有奖活动 | |
---|---|
请大声喊出:我要开发板! | |
【有奖活动】EEPW网站征稿正在进行时,欢迎踊跃投稿啦 | |
【有奖活动】智能可穿戴设备AR/VR如何引领科技新潮流! | |
奖!发布技术笔记,技术评测贴换取您心仪的礼品 | |
【有奖活动】震撼来袭!这场直播将直击工程师的心灵! |
打赏帖 | |
---|---|
如何实现基于NXPiMX.RT1021的BH1730采集被打赏50分 | |
【换取手持数字示波器】AHT10温度检测分享被打赏40分 | |
【换取手持数字示波器】ACM32F070开发板点亮LCD屏和触控按键,串口,ADC被打赏40分 | |
【换取手持数字示波器】ACM32F070LCD屏和触控按键功驱动蜂鸣器分享被打赏40分 | |
【换取手持数字示波器】国民技术PWM功能知识分享被打赏40分 | |
【换取手持数字示波器】放大器运放知识分享被打赏40分 | |
【分享评测,赢取加热台】+开关电源AC输入知识分享被打赏30分 | |
【换取手持数字示波器】N32G430点亮数码管与串口打印、ADC被打赏40分 | |
老胖子聊电路--分析一个反馈电路被打赏50分 | |
【换取手持数字示波器】+点亮WS2812RGB灯被打赏50分 |