共2条
1/1 1 跳转至页
8515,HD44780,ASM 8515和HD44780字符液晶接口的ASM代码
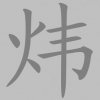
问
;***************************************************************
;Program LCD.ASM
;This Program shows how a LCD with 16 char/ 1 line
;is used with a atmel 8515 and a HD44780 lcd controller
;The crystal is set to 4 MHz
;***************************************************************
.include "8515def.inc"
;Definitions
.equ E = PD0 ;E an Pin PD0
.equ RW = PD1 ;RW an Pin PD1
.equ RS = PD2 ;RS an Pin PD2
.equ BF = PB7 ;Busy flag
;LCD inits
.equ clear_LCD = 0b00000001 ;CLEAR DISPLAY
.equ home_LCD = 0b00000010 ;return home
.equ set_LCD = 0b00111000 ;8 bits,2 line, 5x7 dots
.equ LCD_on = 0b00001110 ;LCD on
.equ entry_mode = 0b00000110 ;set cursor
;Variable definition
.def chr = r0
.def buffer = r16 ;RX/TX Data from LCD
.def counter = r17
.def temp = r18
.def temp2 = r19
.def temp3 = r20
.CSEG
.ORG 0x00 ; Program starts at adress 0x00
rjmp main
;**************************************************************
; Subroutine init
; Init PORTD
;**************************************************************
init: ldi temp,0b11111111 ;PORTD is an output
out DDRD,temp
cbi PORTD,E ;E init
cbi PORTD,RS
cbi PORTD,RW
ret
;***************************************************************
; Subroutine busy_flag
; Test the LCD for sending another instruction
;***************************************************************
busy_flag: ldi temp,0b00000000 ;PORTB is an input
out DDRB,temp
cbi PORTD,RS ;send test
sbi PORTD,RW ;Set LCD in read-mode
sbi PORTD,E ;control LCD
nop
nop
sbic PINB,BF ;LCD busy?
rjmp busy_flag ;no, again
cbi PORTD,E ;disable LCD
ret ;LCD not busy
;***************************************************************
; Subroutine write_data
; Buffer is the data to be sent
;***************************************************************
write_data: rcall busy_flag ;LCD busy?
ldi temp,0b11111111 ;PORTB is an output
out DDRB,temp
sbi PORTD,RS ;Send Data
cbi PORTD,RW ;LCD in write-mode
sbi PORTD,E
out PORTB,buffer ;Send buffer Data
cbi PORTD,E ;disable LCD
ret
;***************************************************************
; Subroutine write_instr
; Sends the instruction for LCD-controller
;***************************************************************
write_instr: rcall busy_flag
ldi temp,0b11111111
out DDRB,temp
cbi PORTD,RS
cbi PORTD,RW
sbi PORTD,E
out PORTB,buffer
cbi PORTD,E
ret
;***************************************************************
; Main
; Text from table is shifted to the right
; **************************************************************
main:
ldi temp,low(RAMEND) ;Initialize stackpointer for parts with SW stack
out SPL,temp
ldi temp,high(RAMEND) ; Commented out since 1200 does not have SRAM
out SPH,temp
rcall init
ldi buffer,set_LCD ;set LCD Function
rcall write_instr
ldi buffer,LCD_on ;LCD on
rcall write_instr
ldi buffer,clear_LCD ;Clear LCD
rcall write_instr
ldi buffer,entry_mode ;Entry mode
rcall write_instr
ldi buffer,0b00000000 ;LCD-Start adress
rcall write_instr
ldi counter,$0F
ldi ZL,LOW(Tabel1*2)
ldi ZH,HIGH(Tabel1*2)
loop_msg1: lpm
mov buffer,chr
rcall write_data
adiw ZL,1
dec counter
brne loop_msg1
ldi buffer,0b11000000
rcall write_instr
ldi counter,$0F
ldi ZL,LOW(Tabel2*2)
ldi ZH,HIGH(Tabel2*2)
loop_msg2: lpm
mov buffer,chr
rcall write_data
adiw ZL,1
no_carry2: dec counter
brne loop_msg2
loop:
ldi temp,0b11111111
out DDRC,temp
ldi buffer,$FF
out PORTC,buffer
ldi buffer,0b00011100
rcall write_instr
rcall delay
rjmp loop
;Tabel1: .DB "123456789abcdef"
Tabel1: .DB "Robot Atmel" ;$00/0F
;Tabel1: .DB "123456789abcdef"
Tabel2: .DB "Fever 1999"
DELAY:
ldi temp,$FF
ldi temp2,$FF
ldi temp3,$09
LOOP_delay:
dec temp
brne LOOP_delay
dec temp2
brne LOOP_delay
dec temp3
brne LOOP_delay
ret
答 1: 好!
;Program LCD.ASM
;This Program shows how a LCD with 16 char/ 1 line
;is used with a atmel 8515 and a HD44780 lcd controller
;The crystal is set to 4 MHz
;***************************************************************
.include "8515def.inc"
;Definitions
.equ E = PD0 ;E an Pin PD0
.equ RW = PD1 ;RW an Pin PD1
.equ RS = PD2 ;RS an Pin PD2
.equ BF = PB7 ;Busy flag
;LCD inits
.equ clear_LCD = 0b00000001 ;CLEAR DISPLAY
.equ home_LCD = 0b00000010 ;return home
.equ set_LCD = 0b00111000 ;8 bits,2 line, 5x7 dots
.equ LCD_on = 0b00001110 ;LCD on
.equ entry_mode = 0b00000110 ;set cursor
;Variable definition
.def chr = r0
.def buffer = r16 ;RX/TX Data from LCD
.def counter = r17
.def temp = r18
.def temp2 = r19
.def temp3 = r20
.CSEG
.ORG 0x00 ; Program starts at adress 0x00
rjmp main
;**************************************************************
; Subroutine init
; Init PORTD
;**************************************************************
init: ldi temp,0b11111111 ;PORTD is an output
out DDRD,temp
cbi PORTD,E ;E init
cbi PORTD,RS
cbi PORTD,RW
ret
;***************************************************************
; Subroutine busy_flag
; Test the LCD for sending another instruction
;***************************************************************
busy_flag: ldi temp,0b00000000 ;PORTB is an input
out DDRB,temp
cbi PORTD,RS ;send test
sbi PORTD,RW ;Set LCD in read-mode
sbi PORTD,E ;control LCD
nop
nop
sbic PINB,BF ;LCD busy?
rjmp busy_flag ;no, again
cbi PORTD,E ;disable LCD
ret ;LCD not busy
;***************************************************************
; Subroutine write_data
; Buffer is the data to be sent
;***************************************************************
write_data: rcall busy_flag ;LCD busy?
ldi temp,0b11111111 ;PORTB is an output
out DDRB,temp
sbi PORTD,RS ;Send Data
cbi PORTD,RW ;LCD in write-mode
sbi PORTD,E
out PORTB,buffer ;Send buffer Data
cbi PORTD,E ;disable LCD
ret
;***************************************************************
; Subroutine write_instr
; Sends the instruction for LCD-controller
;***************************************************************
write_instr: rcall busy_flag
ldi temp,0b11111111
out DDRB,temp
cbi PORTD,RS
cbi PORTD,RW
sbi PORTD,E
out PORTB,buffer
cbi PORTD,E
ret
;***************************************************************
; Main
; Text from table is shifted to the right
; **************************************************************
main:
ldi temp,low(RAMEND) ;Initialize stackpointer for parts with SW stack
out SPL,temp
ldi temp,high(RAMEND) ; Commented out since 1200 does not have SRAM
out SPH,temp
rcall init
ldi buffer,set_LCD ;set LCD Function
rcall write_instr
ldi buffer,LCD_on ;LCD on
rcall write_instr
ldi buffer,clear_LCD ;Clear LCD
rcall write_instr
ldi buffer,entry_mode ;Entry mode
rcall write_instr
ldi buffer,0b00000000 ;LCD-Start adress
rcall write_instr
ldi counter,$0F
ldi ZL,LOW(Tabel1*2)
ldi ZH,HIGH(Tabel1*2)
loop_msg1: lpm
mov buffer,chr
rcall write_data
adiw ZL,1
dec counter
brne loop_msg1
ldi buffer,0b11000000
rcall write_instr
ldi counter,$0F
ldi ZL,LOW(Tabel2*2)
ldi ZH,HIGH(Tabel2*2)
loop_msg2: lpm
mov buffer,chr
rcall write_data
adiw ZL,1
no_carry2: dec counter
brne loop_msg2
loop:
ldi temp,0b11111111
out DDRC,temp
ldi buffer,$FF
out PORTC,buffer
ldi buffer,0b00011100
rcall write_instr
rcall delay
rjmp loop
;Tabel1: .DB "123456789abcdef"
Tabel1: .DB "Robot Atmel" ;$00/0F
;Tabel1: .DB "123456789abcdef"
Tabel2: .DB "Fever 1999"
DELAY:
ldi temp,$FF
ldi temp2,$FF
ldi temp3,$09
LOOP_delay:
dec temp
brne LOOP_delay
dec temp2
brne LOOP_delay
dec temp3
brne LOOP_delay
ret
答 1: 好!
共2条
1/1 1 跳转至页
回复
有奖活动 | |
---|---|
【有奖活动】分享技术经验,兑换京东卡 | |
话不多说,快进群! | |
请大声喊出:我要开发板! | |
【有奖活动】EEPW网站征稿正在进行时,欢迎踊跃投稿啦 | |
奖!发布技术笔记,技术评测贴换取您心仪的礼品 | |
打赏了!打赏了!打赏了! |