共2条
1/1 1 跳转至页
LPC2131,3V,LCM,VDD 请问:用LPC2131开发板3.3V的电源可不可直接连LCM的VDD
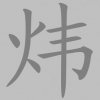
问
请问各位:用LPC2131开发板3.3V的电源可不可直接连LCM的VDD?
现在想用LPC2132来驱动T6963C为控制器的LCM,
一种方式是直接用3.3V的电源来直接连接LCM的VDD,
另外一种方式是用USB口的5V电源来连接LCM的VDD,
而LCM的VEE另外接-5V电源,
两种方式下,LCM就是不亮,郁闷之中。
答 1: 贴上源代码
/* 头文件T6963C.H */
#include "config.h"
#ifndef __T6963_H
#define __T6963_H
#define LCM_RST 1 << 17
#define LCM_WR 1 << 18
#define LCM_RD 1 << 19
#define LCM_CS 1 << 20
#define LCM_CD 1 << 21
#define LCM_DAT (0xff << 18)
/* 定义 LCM复位 */
#define LCM_RESET_H() IO0SET = LCM_RST
#define LCM_RESET_L() IO0CLR = LCM_RST
/* 定义 LCM接口的IO引脚方向 */
#define LCM_CC_DIR() IO0DIR = IO0DIR | (LCM_WR | LCM_RD | LCM_CS | LCM_CD | LCM_RST)
#define LCM_BUS_DIR_IN() IO1DIR = IO1DIR & (~LCM_DAT)
#define LCM_BUS_DIR_OUT() IO1DIR = IO1DIR | LCM_DAT
/* 定义总线起始的GPIO,即D0对应的GPIO值(P1.18) */
#define LCM_BUS 18
/* 输出总线数据宏定义 */
#define LCM_OutData(dat) IO1CLR = 0xff << LCM_BUS; IO1SET = (dat&0xff) << LCM_BUS
/* 读入总线数据宏定义 */
#define LCM_InData() (unsigned char)((IO1PIN & LCM_DAT) >> LCM_BUS)
/* 定义控制信号线电平信号 */
#define SET_LCM_CS() IO0SET = LCM_CS
#define CLR_LCM_CS() IO0CLR = LCM_CS
#define SET_LCM_CD() IO0SET = LCM_CD
#define CLR_LCM_CD() IO0CLR = LCM_CD
#define SET_LCM_RD() IO0SET = LCM_RD
#define CLR_LCM_RD() IO0CLR = LCM_RD
#define SET_LCM_WR() IO0SET = LCM_WR
#define CLR_LCM_WR() IO0CLR = LCM_WR
/* 定义外部函数 */
extern unsigned char LCMReadStatus(unsigned char read_bit);
extern void LCMWriteCmd(unsigned char dat_num,unsigned char dat[2],unsigned char cmd_code);
extern void LCMWriteDat(unsigned char dat);
extern unsigned char LCMRead(void);
extern void LCMInit(void);
extern void LCMClear(void);
#endif
/* T6963C.C */
#include "T6963.h"
/*********************************************************************************
* 名称:DELAY5()
* 功能:软件延时函数。用于LCM显示输出时序控制。
* 入口参数:无
* 出口参数:无
**********************************************************************************/
void DELAY5(void)
{
int i;
for(i=0; i<100; i++);
}
// 读状态位子程序
// 输入: read_bit (0~7)
// 输出: bit(0:disable/no error/display off; 1: enable/error/normal display)
unsigned char LCMReadStatus(unsigned char read_bit)
{
unsigned char status_rd;
CLR_LCM_CS();
SET_LCM_CD();
CLR_LCM_RD();
LCM_BUS_DIR_IN();
DELAY5();
status_rd = LCM_InData(); // LCM data bus
DELAY5();
LCM_BUS_DIR_OUT();
SET_LCM_RD();
if(status_rd & (1 << read_bit))
return 1;
else
return 0;
}
// 写指令和写数据子程序
// 输入: dat_num:参数数目; dat[2]:参数; cmd_code:命令代码
// 输出: 无
void LCMWriteCmd(unsigned char dat_num,unsigned char dat[2],unsigned char cmd_code)
{
unsigned char i;
for(i=0; i<dat_num; dat_num++)
{
while(LCMReadStatus(0) == 0); // 检查命令执行使能
while(LCMReadStatus(1) == 0); // 检查数据读写使能
CLR_LCM_CD(); // CD=0,写数据
DELAY5();
LCM_OutData(dat[i]); // 写入数据
CLR_LCM_WR();
DELAY5();
SET_LCM_WR();
}
while(LCMReadStatus(0) == 0); // 检查命令执行使能
while(LCMReadStatus(1) == 0); // 检查数据读写使能
SET_LCM_CD();
DELAY5();
LCM_OutData(cmd_code); // 写入命令代码
CLR_LCM_WR();
DELAY5();
SET_LCM_WR();
}
// 写数据子程序
// 输入: dat:写入数据
// 输出: 无
void LCMWriteDat(unsigned char dat)
{
CLR_LCM_CD(); // CD=0,写数据
DELAY5();
LCM_OutData(dat); // 写入数据
CLR_LCM_WR();
DELAY5();
SET_LCM_WR();
}
// 读数据子程序
// 输入: 无
// 输出: dat_read: 读入的8位数据
unsigned char LCMRead(void)
{
unsigned char dat_read;
while(LCMReadStatus(0) == 0);
while(LCMReadStatus(1) == 0);
CLR_LCM_CD();
CLR_LCM_RD();
LCM_BUS_DIR_IN();
DELAY5();
dat_read = LCM_InData(); // 读入数据保存在dat_read
DELAY5();
LCM_BUS_DIR_OUT();
SET_LCM_RD();
return dat_read;
}
// 初始化设置子程序
void LCMInit(void)
{
unsigned char dat[2];
LCM_CC_DIR();
LCM_BUS_DIR_OUT();
LCM_RESET_L();
DELAY5();
LCM_RESET_H();
dat[0] = 0x00;
dat[1] = 0x00;
LCMWriteCmd(2,dat,0x40); // 设置文本显示区域首址
dat[0] = 0x20;
dat[1] = 0x00;
LCMWriteCmd(2,dat,0x41); // 设置文本显示区域
dat[0] = 0x00;
dat[1] = 0x08;
LCMWriteCmd(2,dat,0x42); // 设置图形显示区域首址
dat[0] = 0x20;
dat[1] = 0x00;
LCMWriteCmd(2,dat,0x43); // 设置图形显示区域宽度
dat[0] = 0x00;
dat[1] = 0x00;
LCMWriteCmd(2,dat,0x21); // 设置光标指针
dat[0] = 0x00;
dat[1] = 0x00;
LCMWriteCmd(2,dat,0x22); // 设置移位寄存器
dat[0] = 0x00;
dat[1] = 0x00;
LCMWriteCmd(2,dat,0x24); // 设置地址指针
LCMWriteCmd(0,dat,0x92); // 设置闪烁关,光标开
LCMWriteCmd(0,dat,0xa7); // 光标形状设置
LCMWriteCmd(0,dat,0x80); // 显示方式设置 逻辑“或”合成
LCMWriteCmd(0,dat,0x9c); // 显示开关设置 开文本和图形显示
dat[0] = 0x3f;
LCMWriteCmd(1,dat,0xc0); // 写入数据
LCMWriteCmd(1,dat,0xc0);
}
// LCM显示储存区清0
void LCMClear(void)
{
unsigned int i;
unsigned char dat[2];
dat[0] = 0x00;
dat[1] = 0x00;
LCMWriteCmd(2,dat,0x24); // 设置显示储存器首址
LCMWriteCmd(0,dat,0xb0); // 设置自动写方式
for(i=0x2000; i>0; i--)
{
while(LCMReadStatus(3) == 0); // 检查自动写方式使能
LCMWriteDat(0);
}
LCMWriteCmd(0,dat,0xb2);
}
/* 主程序文件 */
#include "config.h"
/********************************************************************************************************
** 函数名称:DelayNS()
** 函数功能:长软件延时
** 入口参数:dly 延时参数,值越大,延时越久
** 出口参数:无
********************************************************************************************************/
void DelayNS(uint32 dly)
{
uint32 i;
for(; dly>0; dly--)
for(i=0; i<50000; i++);
}
/********************************************************************************************************
** 函数名称:main()
** 函数功能:
**
********************************************************************************************************/
uint8 rcv_data;
int main (void)
{
PINSEL0 = 0x00000000; // 所有管脚连接GPIO
PINSEL1 = 0x00000000;
IO0DIR = 0x00000000; // P0所有管脚设置为输出
IO1DIR = 0x00000000; // P1所有管脚设置为输出
IO0CLR = 0x00000000;
IO1CLR = 0x00000000;
LCMInit();
while(1);
return 0;
}
/********************************************************************************************************* 答 2: 跟代码无关。。确认你的LCM是3。3,还是5V。。。 答 3: 两种方式都可以我前一段时间用NIOS,系统是3.3V的,将LCM直接用3.3V供电,可以正常工作,就是显示特别淡,后来改成5V的好了.当时用的内置负压发生器,液晶是HS12864A.
如果连个字影都没有,那么好好看看你的程序和连线. 答 4: T6963电源是5V的,好像也不是宽压的,3V估计不工作 答 5: 楼上的,我已经用USB出来的VDD接在LCM的VDD脚上了楼上的,我已经用USB出来的VDD接在LCM的VDD脚上了,测量是4.76V(资料上写的Vdd最小值为4.5V),应该没问题的。而VEE我接上另外一个+5V电源的GND端,该电源的+5V端与开发板的GND相接,不知道这样子行不行?
运行的时候,LCM_WR、LCM_RD等引脚出来的高电平电压是3.3V,应该逻辑高电平(手册上的Vih最小值=Vdd-2.2=2.8)也没问题。
LCM在负电源接上、拆下的瞬间,会全亮一下,应该还未坏。
在单步运行的时候,
看到读状态位子程序时,读出来的状态数据status_rd的第7位是不确定的。
资料上写着STA7 0:Display off/1:Normal display.
就是说有时候off,有时候正常显示,这是否正常啊?
谢谢~~
答 6: USB接出来再到LCM 这要算算LCM的功率要求多少,USB的供给功率不大的,估计是带不动影起的,你可以外接3。3V到开发板,不用USB的试试看。 答 7: 大家帮我看看初始化设置子程序LCMInit()有没有错误啊?我还不太懂
设置文本显示区域首址、显示区域宽度
设置图形显示区域首址、显示区域宽度
跟
设置移位寄存器、设置地址指针
之间的关系…… 答 8: 最好加括号,小心优先级害人#define LCM_RST (1 << 17)
以此类推...
答 9: 噢,谢谢!不过这个程序应该不关这个的事也许还有些地方没注意到。 答 10: 沉了,顶上去,沉了,顶上去,
不破楼兰终不还~~~
现在想用LPC2132来驱动T6963C为控制器的LCM,
一种方式是直接用3.3V的电源来直接连接LCM的VDD,
另外一种方式是用USB口的5V电源来连接LCM的VDD,
而LCM的VEE另外接-5V电源,
两种方式下,LCM就是不亮,郁闷之中。
答 1: 贴上源代码
/* 头文件T6963C.H */
#include "config.h"
#ifndef __T6963_H
#define __T6963_H
#define LCM_RST 1 << 17
#define LCM_WR 1 << 18
#define LCM_RD 1 << 19
#define LCM_CS 1 << 20
#define LCM_CD 1 << 21
#define LCM_DAT (0xff << 18)
/* 定义 LCM复位 */
#define LCM_RESET_H() IO0SET = LCM_RST
#define LCM_RESET_L() IO0CLR = LCM_RST
/* 定义 LCM接口的IO引脚方向 */
#define LCM_CC_DIR() IO0DIR = IO0DIR | (LCM_WR | LCM_RD | LCM_CS | LCM_CD | LCM_RST)
#define LCM_BUS_DIR_IN() IO1DIR = IO1DIR & (~LCM_DAT)
#define LCM_BUS_DIR_OUT() IO1DIR = IO1DIR | LCM_DAT
/* 定义总线起始的GPIO,即D0对应的GPIO值(P1.18) */
#define LCM_BUS 18
/* 输出总线数据宏定义 */
#define LCM_OutData(dat) IO1CLR = 0xff << LCM_BUS; IO1SET = (dat&0xff) << LCM_BUS
/* 读入总线数据宏定义 */
#define LCM_InData() (unsigned char)((IO1PIN & LCM_DAT) >> LCM_BUS)
/* 定义控制信号线电平信号 */
#define SET_LCM_CS() IO0SET = LCM_CS
#define CLR_LCM_CS() IO0CLR = LCM_CS
#define SET_LCM_CD() IO0SET = LCM_CD
#define CLR_LCM_CD() IO0CLR = LCM_CD
#define SET_LCM_RD() IO0SET = LCM_RD
#define CLR_LCM_RD() IO0CLR = LCM_RD
#define SET_LCM_WR() IO0SET = LCM_WR
#define CLR_LCM_WR() IO0CLR = LCM_WR
/* 定义外部函数 */
extern unsigned char LCMReadStatus(unsigned char read_bit);
extern void LCMWriteCmd(unsigned char dat_num,unsigned char dat[2],unsigned char cmd_code);
extern void LCMWriteDat(unsigned char dat);
extern unsigned char LCMRead(void);
extern void LCMInit(void);
extern void LCMClear(void);
#endif
/* T6963C.C */
#include "T6963.h"
/*********************************************************************************
* 名称:DELAY5()
* 功能:软件延时函数。用于LCM显示输出时序控制。
* 入口参数:无
* 出口参数:无
**********************************************************************************/
void DELAY5(void)
{
int i;
for(i=0; i<100; i++);
}
// 读状态位子程序
// 输入: read_bit (0~7)
// 输出: bit(0:disable/no error/display off; 1: enable/error/normal display)
unsigned char LCMReadStatus(unsigned char read_bit)
{
unsigned char status_rd;
CLR_LCM_CS();
SET_LCM_CD();
CLR_LCM_RD();
LCM_BUS_DIR_IN();
DELAY5();
status_rd = LCM_InData(); // LCM data bus
DELAY5();
LCM_BUS_DIR_OUT();
SET_LCM_RD();
if(status_rd & (1 << read_bit))
return 1;
else
return 0;
}
// 写指令和写数据子程序
// 输入: dat_num:参数数目; dat[2]:参数; cmd_code:命令代码
// 输出: 无
void LCMWriteCmd(unsigned char dat_num,unsigned char dat[2],unsigned char cmd_code)
{
unsigned char i;
for(i=0; i<dat_num; dat_num++)
{
while(LCMReadStatus(0) == 0); // 检查命令执行使能
while(LCMReadStatus(1) == 0); // 检查数据读写使能
CLR_LCM_CD(); // CD=0,写数据
DELAY5();
LCM_OutData(dat[i]); // 写入数据
CLR_LCM_WR();
DELAY5();
SET_LCM_WR();
}
while(LCMReadStatus(0) == 0); // 检查命令执行使能
while(LCMReadStatus(1) == 0); // 检查数据读写使能
SET_LCM_CD();
DELAY5();
LCM_OutData(cmd_code); // 写入命令代码
CLR_LCM_WR();
DELAY5();
SET_LCM_WR();
}
// 写数据子程序
// 输入: dat:写入数据
// 输出: 无
void LCMWriteDat(unsigned char dat)
{
CLR_LCM_CD(); // CD=0,写数据
DELAY5();
LCM_OutData(dat); // 写入数据
CLR_LCM_WR();
DELAY5();
SET_LCM_WR();
}
// 读数据子程序
// 输入: 无
// 输出: dat_read: 读入的8位数据
unsigned char LCMRead(void)
{
unsigned char dat_read;
while(LCMReadStatus(0) == 0);
while(LCMReadStatus(1) == 0);
CLR_LCM_CD();
CLR_LCM_RD();
LCM_BUS_DIR_IN();
DELAY5();
dat_read = LCM_InData(); // 读入数据保存在dat_read
DELAY5();
LCM_BUS_DIR_OUT();
SET_LCM_RD();
return dat_read;
}
// 初始化设置子程序
void LCMInit(void)
{
unsigned char dat[2];
LCM_CC_DIR();
LCM_BUS_DIR_OUT();
LCM_RESET_L();
DELAY5();
LCM_RESET_H();
dat[0] = 0x00;
dat[1] = 0x00;
LCMWriteCmd(2,dat,0x40); // 设置文本显示区域首址
dat[0] = 0x20;
dat[1] = 0x00;
LCMWriteCmd(2,dat,0x41); // 设置文本显示区域
dat[0] = 0x00;
dat[1] = 0x08;
LCMWriteCmd(2,dat,0x42); // 设置图形显示区域首址
dat[0] = 0x20;
dat[1] = 0x00;
LCMWriteCmd(2,dat,0x43); // 设置图形显示区域宽度
dat[0] = 0x00;
dat[1] = 0x00;
LCMWriteCmd(2,dat,0x21); // 设置光标指针
dat[0] = 0x00;
dat[1] = 0x00;
LCMWriteCmd(2,dat,0x22); // 设置移位寄存器
dat[0] = 0x00;
dat[1] = 0x00;
LCMWriteCmd(2,dat,0x24); // 设置地址指针
LCMWriteCmd(0,dat,0x92); // 设置闪烁关,光标开
LCMWriteCmd(0,dat,0xa7); // 光标形状设置
LCMWriteCmd(0,dat,0x80); // 显示方式设置 逻辑“或”合成
LCMWriteCmd(0,dat,0x9c); // 显示开关设置 开文本和图形显示
dat[0] = 0x3f;
LCMWriteCmd(1,dat,0xc0); // 写入数据
LCMWriteCmd(1,dat,0xc0);
}
// LCM显示储存区清0
void LCMClear(void)
{
unsigned int i;
unsigned char dat[2];
dat[0] = 0x00;
dat[1] = 0x00;
LCMWriteCmd(2,dat,0x24); // 设置显示储存器首址
LCMWriteCmd(0,dat,0xb0); // 设置自动写方式
for(i=0x2000; i>0; i--)
{
while(LCMReadStatus(3) == 0); // 检查自动写方式使能
LCMWriteDat(0);
}
LCMWriteCmd(0,dat,0xb2);
}
/* 主程序文件 */
#include "config.h"
/********************************************************************************************************
** 函数名称:DelayNS()
** 函数功能:长软件延时
** 入口参数:dly 延时参数,值越大,延时越久
** 出口参数:无
********************************************************************************************************/
void DelayNS(uint32 dly)
{
uint32 i;
for(; dly>0; dly--)
for(i=0; i<50000; i++);
}
/********************************************************************************************************
** 函数名称:main()
** 函数功能:
**
********************************************************************************************************/
uint8 rcv_data;
int main (void)
{
PINSEL0 = 0x00000000; // 所有管脚连接GPIO
PINSEL1 = 0x00000000;
IO0DIR = 0x00000000; // P0所有管脚设置为输出
IO1DIR = 0x00000000; // P1所有管脚设置为输出
IO0CLR = 0x00000000;
IO1CLR = 0x00000000;
LCMInit();
while(1);
return 0;
}
/********************************************************************************************************* 答 2: 跟代码无关。。确认你的LCM是3。3,还是5V。。。 答 3: 两种方式都可以我前一段时间用NIOS,系统是3.3V的,将LCM直接用3.3V供电,可以正常工作,就是显示特别淡,后来改成5V的好了.当时用的内置负压发生器,液晶是HS12864A.
如果连个字影都没有,那么好好看看你的程序和连线. 答 4: T6963电源是5V的,好像也不是宽压的,3V估计不工作 答 5: 楼上的,我已经用USB出来的VDD接在LCM的VDD脚上了楼上的,我已经用USB出来的VDD接在LCM的VDD脚上了,测量是4.76V(资料上写的Vdd最小值为4.5V),应该没问题的。而VEE我接上另外一个+5V电源的GND端,该电源的+5V端与开发板的GND相接,不知道这样子行不行?
运行的时候,LCM_WR、LCM_RD等引脚出来的高电平电压是3.3V,应该逻辑高电平(手册上的Vih最小值=Vdd-2.2=2.8)也没问题。
LCM在负电源接上、拆下的瞬间,会全亮一下,应该还未坏。
在单步运行的时候,
看到读状态位子程序时,读出来的状态数据status_rd的第7位是不确定的。
资料上写着STA7 0:Display off/1:Normal display.
就是说有时候off,有时候正常显示,这是否正常啊?
谢谢~~
答 6: USB接出来再到LCM 这要算算LCM的功率要求多少,USB的供给功率不大的,估计是带不动影起的,你可以外接3。3V到开发板,不用USB的试试看。 答 7: 大家帮我看看初始化设置子程序LCMInit()有没有错误啊?我还不太懂
设置文本显示区域首址、显示区域宽度
设置图形显示区域首址、显示区域宽度
跟
设置移位寄存器、设置地址指针
之间的关系…… 答 8: 最好加括号,小心优先级害人#define LCM_RST (1 << 17)
以此类推...
答 9: 噢,谢谢!不过这个程序应该不关这个的事也许还有些地方没注意到。 答 10: 沉了,顶上去,沉了,顶上去,
不破楼兰终不还~~~
共2条
1/1 1 跳转至页