- #include "stm32f10x.h"
- #include "stm32_eval.h"
- #include
- volatile int flag;
- #define Set_B20() GPIO_SetBits(GPIOC, GPIO_Pin_12) //上拉关闭PC12
- #define Reset_B20() GPIO_ResetBits(GPIOC, GPIO_Pin_12) //下拉打开PC12
- #define Read_B20() GPIO_ReadInputDataBit(GPIOC,GPIO_Pin_12) //读PC12状态
- unsigned char Error_Flag=0;
- unsigned char zf=0;
- void SysTick_Configuration(void)
- {
- /* Setup SysTick Timer for 10 msec interrupts */
- if (SysTick_Config(48000)) //SysTick配置
- {
- /* Capture error */
- while (1);
- }
- /* Configure the SysTick handler priority */
- NVIC_SetPriority(SysTick_IRQn, 0x0); //SysTick中断优先级
- }
- /** @addtogroup STM32F10x_StdPeriph_Examples
- * @{
- */
- /** @addtogroup EXTI_Config
- * @{
- */
- /* Private typedef -----------------------------------------------------------*/
- /* Private define ------------------------------------------------------------*/
- /* Private macro -------------------------------------------------------------*/
- /* Private variables ---------------------------------------------------------*/
- GPIO_InitTypeDef GPIO_InitStructure; //结构体的命名
- USART_InitTypeDef USART_InitStructure; //结构体的命名
- USART_ClockInitTypeDef USART_ClockInitStructure; //结构体的命名
- void RCC_Configuration(void)
- {
- RCC_DeInit(); //将外设RCC的所有寄存器重新设为缺省值
- RCC_HSICmd(ENABLE); //使能内部高速晶振
- while(RCC_GetFlagStatus(RCC_FLAG_HSIRDY) == RESET); //当SHI晶振就绪则重新设定
- RCC_SYSCLKConfig(RCC_SYSCLKSource_HSI); //设置系统时钟,选择SHI时钟为系统时钟
- RCC_HSEConfig(RCC_HSE_OFF); //设置外部高速晶振,HSE晶振OFF
- RCC_LSEConfig(RCC_LSE_OFF); //设置外部低速晶振,LSE晶振OFF
- //******配置PLL时钟频率为48MHZ*******//
- RCC_PLLConfig(RCC_PLLSource_HSI_Div2,RCC_PLLMul_8); //RCC_PLLMul_x 即设置PLL时钟频率为 6*x MHz
- //************************************//
- RCC_PLLCmd(ENABLE); ////*******************使能PLL
- while(RCC_GetFlagStatus(RCC_FLAG_PLLRDY) == RESET); //PLL就绪
- RCC_ADCCLKConfig(RCC_PCLK2_Div4); // ADC时钟=PCLK/2
- RCC_PCLK2Config(RCC_HCLK_Div1); // APB2时钟=HCLK
- RCC_PCLK1Config(RCC_HCLK_Div2); /// APB1时钟=HCLK/2
- RCC_HCLKConfig(RCC_SYSCLK_Div1); // AHB时钟=系统时钟
- RCC_SYSCLKConfig(RCC_SYSCLKSource_PLLCLK); // 选择PLL为系统时钟
- while(RCC_GetSYSCLKSource() != 0x08); //当PLL不是系统时钟
- // SystemInit();
- RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOD|RCC_APB2Periph_AFIO, ENABLE); //使能APB2外设时钟/****GPIOD时钟和功能复用IO时钟***/
- GPIO_PinRemapConfig(GPIO_Remap_SWJ_JTAGDisable,ENABLE);//disable JTAG SW_DP使能
- RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOD|RCC_APB2Periph_AFIO, ENABLE);
- GPIO_PinRemapConfig(GPIO_Remap_SWJ_JTAGDisable,ENABLE);//disable JTAG
- GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2; // //选择设置GPIO管脚
- GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; ////设置管脚速率
- GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; ////设置管脚工作状态,此为推挽输出
- GPIO_Init(GPIOD, &GPIO_InitStructure); //初始化GPIOD
- GPIO_ResetBits(GPIOD,GPIO_Pin_2); //上拉关闭蜂鸣器
- RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC|RCC_APB2Periph_AFIO, ENABLE);
- GPIO_PinRemapConfig(GPIO_Remap_SWJ_JTAGDisable,ENABLE);//disable JTAG
- GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0|GPIO_Pin_1|GPIO_Pin_2|GPIO_Pin_3|GPIO_Pin_4|GPIO_Pin_5|GPIO_Pin_6|GPIO_Pin_7;//LED
- GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
- GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
- GPIO_Init(GPIOC, &GPIO_InitStructure);
- GPIO_SetBits(GPIOC,GPIO_Pin_0|GPIO_Pin_1|GPIO_Pin_2|GPIO_Pin_3|GPIO_Pin_4|GPIO_Pin_5|GPIO_Pin_6|GPIO_Pin_7); // GPIOC.0到GPIOC.7输出胃叩缙姜
- RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE); //使能TIM2时钟
- }
- void USART_int(long BaudRate)
- {
- RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA|RCC_APB2Periph_USART1,ENABLE);//使能GPIOA、USART1外设时钟
- GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
- GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; //GPIO的输出速率为50MHz
- GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
- GPIO_Init(GPIOA, &GPIO_InitStructure);
- /* PA10 USART1_Rx */
- GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
- GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING; //使能外设GPIOC端口时钟
- GPIO_Init(GPIOA, &GPIO_InitStructure);
- /* USARTx configured as follow:
- - BaudRate = 115200 baud
- - Word Length = 8 Bits
- - One Stop Bit
- - No parity
- - Hardware flow control disabled (RTS and CTS signals)
- - Receive and transmit enabled
- */
- USART_InitStructure.USART_BaudRate = BaudRate;//设置USART传输波特率 BaudRate = 9600 可以直接写9600
- USART_InitStructure.USART_WordLength = USART_WordLength_8b;//一帧传输或者接收的数据位数为8bit
- USART_InitStructure.USART_StopBits = USART_StopBits_1;//在帧结尾传输一个停止位
- USART_InitStructure.USART_Parity = USART_Parity_No;//奇偶模式失能
- USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;//硬件流控制失能
- USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;//使能接收发模式
- USART_ClockInitStructure.USART_Clock = USART_Clock_Disable; //时钟低电平活动
- USART_ClockInitStructure.USART_CPOL = USART_CPOL_Low; //引脚时钟输出低电平时钟
- USART_ClockInitStructure.USART_CPHA = USART_CPHA_2Edge; //第二个时钟边沿开始捕获数据
- USART_ClockInitStructure.USART_LastBit = USART_LastBit_Disable;//最后一位数据的时钟脉冲不从SCLK输出
- USART_ClockInit(USART1, &USART_ClockInitStructure); //引用结构体的成员
- USART_Init(USART1, &USART_InitStructure);//USART1初始化
- USART_Cmd(USART1, ENABLE);//使能USART1时钟外设
- USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);//使能接受中断
- USART_Cmd(USART1, ENABLE); //使能 USART
- }
- void delay_18b20(u32 nus) //18b20按照严格的时序工作,这是特定的一个延时函数(自定义)
- {
- u16 i;
- while(nus--)
- for(i=12;i>0;i--);
- }
- void Init18B20(void) //18B20初始化
- {
- u8 aa=0;
- u8 count =0;
- RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);//使能PC时钟
- GPIO_InitStructure.GPIO_Pin = GPIO_Pin_12; //配置端口GPIOC.12
- GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_OD;//开漏输出
- GPIO_Init(GPIOC, &GPIO_InitStructure); //引用结构体的变量
- Set_B20() ; // GPIO_SetBits(GPIOC, GPIO_Pin_12)
- delay_18b20(1);
- Reset_B20(); //重置18B20
- delay_18b20(480);
- Set_B20();
- // delay_18b20(500);
- delay_18b20(480);
- count=0;
- aa=Read_B20(); //温度读取
- /****个人认为限制温度不超过99度,作为一个保护*/
- while(!aa && count<100) //判断aa的非和计数器count的值是否都小于100
- {
- aa=Read_B20(); //
- count++; //count自加1
- }
- if(count>=99)
- Error_Flag=1; //错误返回值1
- else
- Error_Flag=0; //错误返回值0
- }
- unsigned char Read18B20(void)//按位读取数据
- {
- unsigned char i=0;
- unsigned char date=0;
- u8 tempp;
- for(i=8;i>0;i--)
- {
- Reset_B20(); //打开PC12
- date>>=1; //标志右移一位
- delay_18b20(1);
- Set_B20(); //关闭
- delay_18b20(1);
- tempp=Read_B20(); //读取温度
- if(tempp) //判断tempp是否为1
- date|=0x80; // 1000 0000 将最高位填1 ,然后右移,每次最高位由0变1,使8位全部传递完毕 0xff = 1111 1111
- delay_18b20(60); //延时
- }
- return(date); //返回值是无符号的字符型的类型 date
- }
- void Write18B20(unsigned char date)//向18b20写数据
- {
- unsigned char i=0;
- for (i=8; i>0; i--)
- {
- Reset_B20();
- delay_18b20(1);
- if(date & 0x01)
- {
- Set_B20();
- }
- else
- { Reset_B20();}
- delay_18b20(60);
- date>>=1;
- Set_B20();
- delay_18b20(1);
- }
- delay_18b20(15);
- }
- float Read_T()//读温度
- {
- unsigned char TUp,TDown;
- unsigned char fTemp;
- u8 TT=0;
- float Temp = 0;
- Init18B20();
- Write18B20(0xcc);
- Write18B20(0x44);
- Init18B20();
- Write18B20(0xcc);
- Write18B20(0xbe);
- TDown = Read18B20();
- TUp = Read18B20();
- if(TUp>0x7f)
- {
- TDown=~TDown;
- TUp=~TUp+1;
- TUp/=8;
- zf=1;
- }
- else
- zf=0;
- fTemp=TDown&0x0f;
- TUp<<=4;
- TDown>>=4;
- TT=TUp|TDown;
- Temp=TT+(float)fTemp/16;
- return(Temp);
- }
- int main(void)
- {
- /*!< At this stage the microcontroller clock setting is already configured,
- this is done through SystemInit() function which is called from startup
- file (startup_stm32f10x_xx.s) before to branch to application main.
- To reconfigure the default setting of SystemInit() function, refer to
- system_stm32f10x.c file
- */
- /* System Clocks Configuration */
- char ID[10]; //定义一个字符型数组,长度为10
- int i;
- RCC_Configuration(); //配置RCC时钟
- USART_int(115200); //波特率的设置
- SysTick_Configuration(); //系统滴答时钟的调用
- printf(" config done...\r\n"); //打印输出
- //delay_ms(1000);
- Init18B20(); //初始化18b20;
- Write18B20(0x34); //写入读取地址的命令
- delay_18b20(20); //延时
- while(1)
- {
- if(flag == 300) //判断flag是否等于300
- {
- printf(" the ID is:") ;
- for(i=0;i<8;i++) //字符不能直接输出,要按位输出
- {
- ID[i] = Read18B20();//读取地址
- printf("%d", ID[i]);//输出地址
- }
- printf("\r\n") ; //换行
- }
- if(flag == 500) //判断flag是否等于500
- {
- printf("The Temperature is:%f\r\n",Read_T());//读取温度并输出
- printf("===================================================\r\n");
- }
- }
- }
- #ifdef USE_FULL_ASSERT
- /**
- * @brief Reports the name of the source file and the source line number
- * where the assert_param error has occurred.
- * @param file: pointer to the source file name
- * @param line: assert_param error line source number
- * @retval None
- */
- void assert_failed(uint8_t* file, uint32_t line)
- {
- /* User can add his own implementation to report the file name and line number,
- ex: printf("Wrong parameters value: file %s on line %d\r\n", file, line) */
- /* Infinite loop */
- while (1)
- {
- }
- }
- #endif
- /**
- * @}
- */
- /**
- * @}
- */
- #ifdef __GNUC__
- /* With GCC/RAISONANCE, small printf (option LD Linker->Libraries->Small printf
- set to 'Yes') calls __io_putchar() */
- #define PUTCHAR_PROTOTYPE int __io_putchar(int ch)
- #else
- #define PUTCHAR_PROTOTYPE int fputc(int ch, FILE *f)
- #endif /* __GNUC__ */
- /**
- * @brief Retargets the C library printf function to the USART.
- * @param None
- * @retval None
- */
- PUTCHAR_PROTOTYPE
- {
- /* Place your implementation of fputc here */
- /* e.g. write a character to the USART */
- USART_SendData(EVAL_COM1, (uint8_t) ch);
- /* Loop until the end of transmission */
- while (USART_GetFlagStatus(EVAL_COM1, USART_FLAG_TC) == RESET)
- {}
- return ch;
- }
- #ifdef USE_FULL_ASSERT
- /**
- * @brief Reports the name of the source file and the source line number
- * where the assert_param error has occurred.
- * @param file: pointer to the source file name
- * @param line: assert_param error line source number
- * @retval None
- */
- void assert_failed(uint8_t* file, uint32_t line)
- {
- /* User can add his own implementation to report the file name and line number,
- ex: printf("Wrong parameters value: file %s on line %d\r\n", file, line) */
- /* Infinite loop */
- while (1)
- {
- }
- }
- #endif
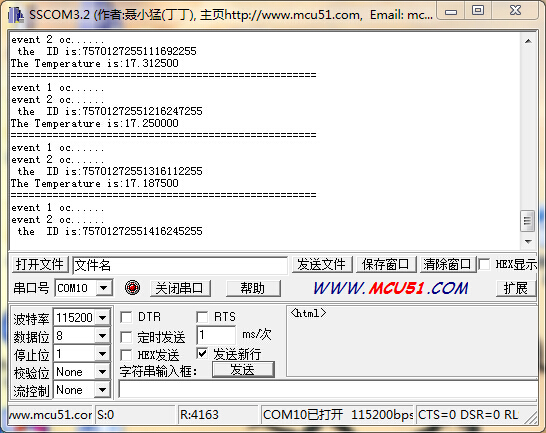
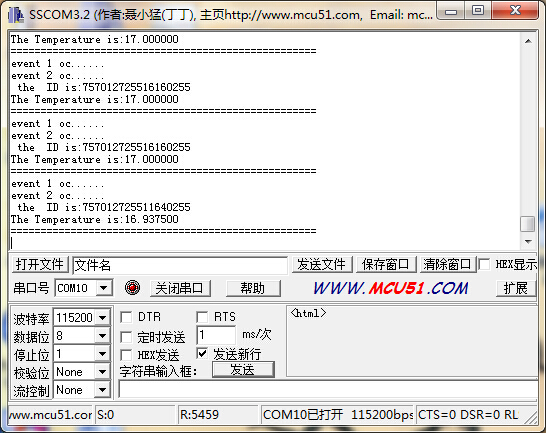