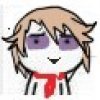
除了LCD显示,读取模拟值(湿度传感器)以外,还要控制继电器
这个就更简单了,就是给高电平或者低电平就可以啦
// Demo for Grove - Starter Kit V2.0 // Uses the Grove - Button to control the Grove - Relay. // When the button is pressed, the relay will be in the "closed" position. // Connect the Grove - Button to the socket marked D3 // Connect the Grove - Relay to D8 // Define the pins to which the button and relay are attached. const int buttonPin = 3; const int relayPin = 8; void setup() { // Configure the relay's pin for output signals. pinMode(relayPin, OUTPUT); // Configure the button's pin for input signals. pinMode(buttonPin, INPUT); } void loop() { // Read the state of the button. int buttonState = digitalRead(buttonPin); // If the button is pressed, activate (close) the relay. if (buttonState == 1) { digitalWrite(relayPin, HIGH); } else { digitalWrite(relayPin, LOW); } delay(10); }
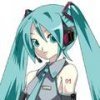
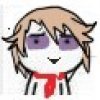
/* Web client This sketch connects to a website (http://www.google.com) using an Arduino Wiznet Ethernet shield. Circuit: * Ethernet shield attached to pins 10, 11, 12, 13 created 18 Dec 2009 modified 9 Apr 2012 by David A. Mellis */ #include <SPI.h> #include <Ethernet.h> // Enter a MAC address for your controller below. // Newer Ethernet shields have a MAC address printed on a sticker on the shield byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED }; IPAddress server(221,200,245,242); // Google // Initialize the Ethernet client library // with the IP address and port of the server // that you want to connect to (port 80 is default for HTTP): EthernetClient client; void setup() { // Open serial communications and wait for port to open: Serial.begin(9600); while (!Serial) { ; // wait for serial port to connect. Needed for Leonardo only } // start the Ethernet connection: if (Ethernet.begin(mac) == 0) { Serial.println("Failed to configure Ethernet using DHCP"); // no point in carrying on, so do nothing forevermore: for(;;) ; } // give the Ethernet shield a second to initialize: delay(1000); Serial.println("connecting..."); // if you get a connection, report back via serial: if (client.connect(server, 80)) { Serial.println("connected"); // Make a HTTP request: client.println("GET / HTTP/1.0"); client.println(); } else { // if you didn't get a connection to the server: Serial.println("connection failed"); } } void loop() { // if there are incoming bytes available // from the server, read them and print them: if (client.available()) { char c = client.read(); Serial.print(c); } // if the server's disconnected, stop the client: if (!client.connected()) { Serial.println(); Serial.println("disconnecting."); client.stop(); // do nothing forevermore: for(;;) ; } }
使用usb以太网的网络客户端
因为google被封锁,所以我将IP换成了我自己搭建的服务器IP
返回内容如下:
HTTP/1.1 200 OK Date: Fri, 09 Sep 2016 01:04:32 GMT Server: Apache/2.4.10 (Raspbian) Last-Modified: Sat, 11 Jun 2016 00:44:09 GMT ETag: "d-534f5f40499a6" Accept-Ranges: bytes Content-Length: 13 Connection: close Content-Type: text/html Hello World!
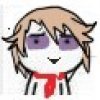
/* Web client This sketch connects to a website (http://www.google.com) using a WiFi shield. This example is written for a network using WPA encryption. For WEP or WPA, change the Wifi.begin() call accordingly. This example is written for a network using WPA encryption. For WEP or WPA, change the Wifi.begin() call accordingly. Circuit: * WiFi shield attached created 13 July 2010 by dlf (Metodo2 srl) modified 31 May 2012 by Tom Igoe */ #include #include char ssid[] = "MYWIFI"; // your network SSID (name) char pass[] = "xxxxxx"; // your network password (use for WPA, or use as key for WEP) int keyIndex = 0; // your network key Index number (needed only for WEP) int status = WL_IDLE_STATUS; // if you don't want to use DNS (and reduce your sketch size) // use the numeric IP instead of the name for the server: //IPAddress server(74,125,232,128); // numeric IP for Google (no DNS) char server[] = "iot.godpub.com"; // name address for Google (using DNS) // Initialize the Ethernet client library // with the IP address and port of the server // that you want to connect to (port 80 is default for HTTP): WiFiClient client; void setup() { //Initialize serial and wait for port to open: Serial.begin(9600); while (!Serial) { ; // wait for serial port to connect. Needed for Leonardo only } // check for the presence of the shield: if (WiFi.status() == WL_NO_SHIELD) { Serial.println("WiFi shield not present"); // don't continue: while(true); } String fv = WiFi.firmwareVersion(); if( fv != "1.1.0" ) Serial.println("Please upgrade the firmware"); // attempt to connect to Wifi network: while (status != WL_CONNECTED) { Serial.print("Attempting to connect to SSID: "); Serial.println(ssid); // Connect to WPA/WPA2 network. Change this line if using open or WEP network: status = WiFi.begin(ssid, pass); // wait 10 seconds for connection: delay(10000); } Serial.println("Connected to wifi"); printWifiStatus(); Serial.println("\nStarting connection to server..."); // if you get a connection, report back via serial: if (client.connect(server, 80)) { Serial.println("connected to server"); // Make a HTTP request: client.println("GET / HTTP/1.1"); client.println("Host: iot.godpub.com"); client.println("Connection: close"); client.println(); } } void loop() { // if there are incoming bytes available // from the server, read them and print them: while (client.available()) { char c = client.read(); Serial.write(c); } // if the server's disconnected, stop the client: if (!client.connected()) { Serial.println(); Serial.println("disconnecting from server."); client.stop(); // do nothing forevermore: while(true); } } void printWifiStatus() { // print the SSID of the network you're attached to: Serial.print("SSID: "); Serial.println(WiFi.SSID()); // print your WiFi shield's IP address: IPAddress ip = WiFi.localIP(); Serial.print("IP Address: "); Serial.println(ip); // print the received signal strength: long rssi = WiFi.RSSI(); Serial.print("signal strength (RSSI):"); Serial.print(rssi); Serial.println(" dBm"); }
因为USB以太网需要连接电脑,而实际应用中,链接电脑不太现实
不能为了自动浇花配置个电脑
所以使用WIFI还是比较方便的
其中"MYWIFI"是我的无线网ID
"xxxxxx"是我的无线网密码
iot.godpub.com 是我自建的web服务器域名
串口返回的全部信息如下:
Attempting to connect to SSID: MYWIFI Connected to wifi SSID: MYWIFI IP Address: 192.168.249.243 signal strength (RSSI):-40 dBm Starting connection to server... connected to server HTTP/1.1 200 OK Date: Fri, 09 Sep 2016 01:04:32 GMT Server: Apache/2.4.10 (Raspbian) Last-Modified: Sat, 11 Jun 2016 00:44:09 GMT ETag: "d-534f5f40499a6" Accept-Ranges: bytes Content-Length: 13 Connection: close Content-Type: text/html Hello World!
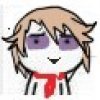
网络数据发送
温湿度以及是否灌溉信息定时通过HTTP协议上报至网络。
GET请求格式大致如下:
/water.php?h=222&t=28.2&s=1
其中:
H为湿度
T为温度
s为是否执行灌溉
详见代码。
服务器端
服务器端应该实现的功能包括但不限于:
接收并存储数据到数据库
提供展示页面以表格或者其它直观的方式展示数据。
本例中我使用BANAPI M2+ 自建了LMAP网站环境。
使用PHP接收数据。
因为服务器端属于另外的范畴,本例不做深入讨论。
服务器端最简单的示例代码如下:
<?php printf("Humidity:\t%.2f\n", $_GET['h']); printf("Temperature:\t%.2f\n", $_GET['t']); $status = $_GET['s']==0?"False":"True"; printf("Watered:\t%s\n", $status); ?>
主要作用为将Edison发送的数据以一定格式显示。
可用于调试Edison发送网络数据部分。
回复
有奖活动 | |
---|---|
发原创文章 【每月瓜分千元赏金 凭实力攒钱买好礼~】 | |
【EEPW在线】E起听工程师的声音! | |
“我踩过的那些坑”主题活动——第001期 | |
高校联络员开始招募啦!有惊喜!! | |
【工程师专属福利】每天30秒,积分轻松拿!EEPW宠粉打卡计划启动! | |
送您一块开发板,2025年“我要开发板活动”又开始了! | |
打赏了!打赏了!打赏了! |
打赏帖 | |
---|---|
【我踩过的那些坑】电感选型错误导致的处理器连接不上被打赏50分 | |
【我踩过的那些坑】工作那些年踩过的记忆深刻的坑被打赏10分 | |
【我踩过的那些坑】DRC使用位置错误导致的问题被打赏100分 | |
我踩过的那些坑之混合OTL功放与落地音箱被打赏50分 | |
汽车电子中巡航控制系统的使用被打赏10分 | |
【我踩过的那些坑】工作那些年踩过的记忆深刻的坑被打赏100分 | |
分享汽车电子中巡航控制系统知识被打赏10分 | |
分享安全气囊系统的检修注意事项被打赏10分 | |
分享电子控制安全气囊计算机知识点被打赏10分 | |
【分享开发笔记,赚取电动螺丝刀】【OZONE】使用方法总结被打赏20分 |